Android configuration
Upon installation of this plugin, configuration is needed on Android before running the project again. If this is not done, an error of No implementation found would show because the Facebook SDK on Android would throw an Exception error if the configuration is not yet defined. This error also locks the other plugins in your project, so if the plugin is not yet needed, either remove it or comment it out from the pubspec.yaml file.
Go to Facebook Login for Android - Quickstart
- Select an App or Create a New App
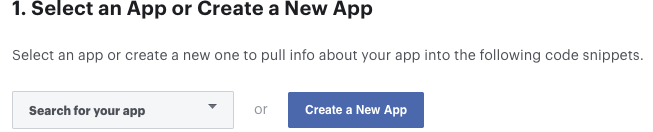
Skip the step 2 (Download the Facebook App)
Skip the step 3 (Integrate the Facebook SDK)
Edit Your Resources and Manifest add this config in your android project
Open your
/android/app/src/main/res/values/strings.xml
file, or create one if it doesn't exists.Add the following (replace
{your-app-id}
with your facebook app Id) and with your client token:<string name="facebook_app_id">{your-app-id}</string>
<string name="facebook_client_token">YOUR_CLIENT_TOKEN</string>Here one example of
strings.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="facebook_app_id">1365719610250300</string>
<string name="facebook_client_token">YOUR_CLIENT_TOKEN</string>
</resources>You can find the client token in your facebook developers console
Open the
/android/app/src/main/AndroidManifest.xml
file.Add the following uses-permission element after the application element
<uses-permission android:name="android.permission.INTERNET"/>
Add the following meta-data element, an activity for Facebook, and an activity and intent filter for Chrome Custom Tabs inside your application element
<meta-data android:name="com.facebook.sdk.ApplicationId" android:value="@string/facebook_app_id"/>
<meta-data android:name="com.facebook.sdk.ClientToken" android:value="@string/facebook_client_token"/>Here one example of
AndroidManifest.xml
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="app.meedu.flutter_facebook_auth_example">
<uses-permission android:name="android.permission.INTERNET" />
<application
android:name="${applicationName}"
android:icon="@mipmap/ic_launcher"
android:label="facebook auth">
<meta-data
android:name="com.facebook.sdk.ApplicationId"
android:value="@string/facebook_app_id" />
<meta-data
android:name="com.facebook.sdk.ClientToken"
android:value="@string/facebook_client_token"/>
<activity
android:name=".MainActivity"
android:configChanges="orientation|keyboardHidden|keyboard|screenSize|smallestScreenSize|locale|layoutDirection|fontScale|screenLayout|density|uiMode"
android:hardwareAccelerated="true"
android:launchMode="singleTop"
android:theme="@style/LaunchTheme"
android:exported="true"
android:windowSoftInputMode="adjustResize">
<meta-data
android:name="io.flutter.embedding.android.NormalTheme"
android:resource="@style/NormalTheme" />
<meta-data
android:name="io.flutter.embedding.android.SplashScreenDrawable"
android:resource="@drawable/launch_background" />
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<meta-data
android:name="flutterEmbedding"
android:value="2" />
</application>
</manifest>
Associate Your Package Name and Default Class with Your App
- Provide the Development and Release Key Hashes for Your App
To find info to how to generate you key hash go to https://developers.facebook.com/docs/facebook-login/android?locale=en_US#6--provide-the-development-and-release-key-hashes-for-your-app
Note: if your application uses Google Play App Signing then you should get certificate SHA-1 fingerprint from Google Play Console and convert it to base64
You should add key hashes for every build variants like release, debug, CI/CD, etc.
- Queries Apps that target Android API 30+ (Android 11+) cannot call Facebook native apps unless the package visibility needs are declared. Please follow https://developers.facebook.com/docs/android/troubleshooting/#faq_267321845055988 to make the declaration. To solve it you have to add in the AndroidManifest.xml file:
<manifest package="com.example.app">
<queries>
<provider android:authorities="com.facebook.katana.provider.PlatformProvider" />
</queries>
...
</manifest>